- 開発技術
Django Rest framework simple JWT認証関連
- Django
この記事を書いたチーム:frontier
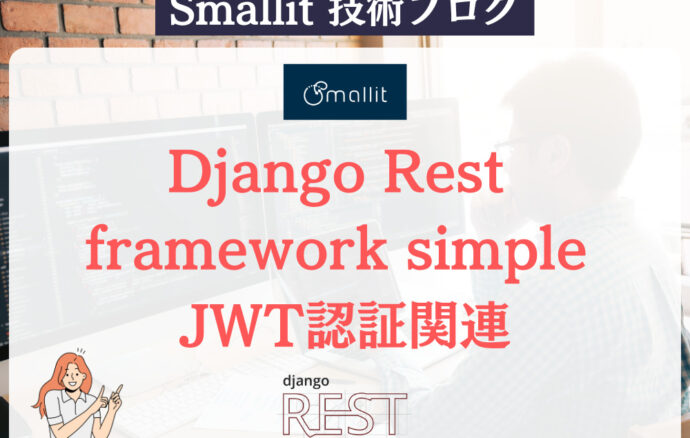
目次
Django Rest framework simple JWTとは
【エンジニア募集中】フルリモート可◎、売上/従業員数9年連続UP、平均残業8時間、有給取得率90%、年休124日以上 etc. 詳細はこちらから>
Django Rest Frameworkのsimple JWTは、JSONWebTokensを使ってAPIの認証を行うための便利なパッケージです。JSONWebTokensは、ユーザーの認証情報をJSONフォーマットで表現したものです。
simple JWTの主な特徴は以下の通りです。
トークンベースの認証:
ユーザーはJWTトークンを使って認証を行います。このトークンはリクエストのヘッダーに含められます。
ステートレス:
JWTはサーバー側で状態を保持する必要がありません。トークン自体にユーザー情報が含まれているため。
期限切れ対策:
トークンには有効期限が設定できます。有効期限を過ぎると、新しいトークンを要求する必要があります。
クライアントとサーバーの疎通用トークン:
アクセストークンとリフレッシュトークンの2種類のトークンが用意されています。
Simple JWTのインストール
pip install djangorestframework-simplejwt
settings.py の設定を追加/更新
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
INSTALLED_APPS = [ ... 'rest_framework', 'rest_framework_simplejwt', ] REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': ( 'rest_framework_simplejwt.authentication.JWTAuthentication', # Use JWT Authentication ), } # Simple JWT settings SIMPLE_JWT = { 'ACCESS_TOKEN_LIFETIME': timedelta(days=1), 'REFRESH_TOKEN_LIFETIME': timedelta(days=1), 'ROTATE_REFRESH_TOKENS': False, 'BLACKLIST_AFTER_ROTATION': False, 'ALGORITHM': 'HS256', 'SIGNING_KEY': SECRET_KEY, 'VERIFYING_KEY': None, 'AUTH_HEADER_TYPES': ('Bearer',), 'USER_ID_FIELD': 'username', 'USER_ID_CLAIM': 'username', 'AUTH_TOKEN_CLASSES': ('rest_framework_simplejwt.tokens.AccessToken',), 'TOKEN_TYPE_CLAIM': 'token_type', } |
カスタマイズのJWT認証作成
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
class CacheJWTAuthentication(JWTAuthentication): """ Custom JWT Authentication class that uses Redis cache for token validation. """ def get_user(self, validated_token): """ Retrieves the user associated with the given validated token. """ try: username = validated_token.get('username') access_token = validated_token if not username or not access_token: raise TokenValidationError("Username or access token is missing") # check if token in cache token_cache_key = f"{TOKEN_CACHE_PREFIX}{access_token}" if not cache.get(token_cache_key): raise TokenValidationError("Access token not found in cache") # read user from cache cache_key = f"{USER_CACHE_PREFIX}{username}" user_data = cache.get(cache_key) if not user_data: # get user from database user = super().get_user(validated_token) if user: # save user information to cache save_user(user) save_roles(user) save_permissions(user) return user else: raise UserDataError("User data not found") else: user = TUser(**user_data) return user except RedisError as e: raise CacheError(f"Redis error occurred: {e}") except OperationalError as e: raise DatabaseError(f"Database operational error: {e}") except Exception as e: raise Exception(f"Unexpected error in CacheJWTAuthentication: {e}") |
views.pyにimportする
1 2 3 |
from rest_framework_simplejwt.tokens import RefreshToken from rest_framework_simplejwt.authentication import JWTAuthentication from .utils.cache_JWTAuthentication import CacheJWTAuthentication |
JWT認証を使ってログイン処理
1 2 3 |
refresh = RefreshToken.for_user(user) refresh_token = str(refresh) access_token = str(refresh.access_token) |
APIviewsに認証classを設定
1 2 3 4 5 |
class MyExampleView(APIView): authentication_classes = [CacheJWTAuthentication] ... def post(self, request): ... |
Headerの設定
1 2 3 |
{ "Authorization" : "Bearer ${TOKEN}" } |
これに対してfrontendの修正が必要です。
【エンジニア募集中】フルリモートも◎(リモート率85.7%)、平均残業8時間、年休124日以上、有給取得率90% etc. 詳細はこちらから>